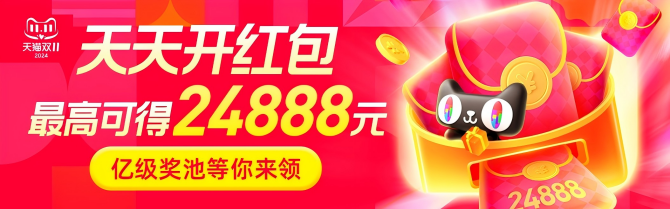
SpringAOP入门基础银行转账实例————事务处理
AOP为Aspect Oriented Programming 的缩写,意思为面向切面编程,是通过编译方式和运行期动态代理实现程序功能的统一维护的一种技术。
AOP编程思想
AOP面向切面是一种编程思想,是oop的延续,是软件开发中的一个热点,也是Spring框架中的一个重要的内容,是函数式编程的一种衍生泛型。利用AOP可以对业务逻辑的各个部门进行隔离,从而使得业务逻辑各个部分之间的耦合度降低,提高程序的可重用性,同时提高了开发的效率。
AOP得应用场景:
事务管理
权限校验
日志记录
性能监控
Spring 中常用的术语
Joinpoint(连接点) :所谓连接点是指那些被拦截到的点。在spring中,这些点指的是方法,因为spring只支持方法类型的连接点
Pointcut(切入点):所谓切入点是指我们要对哪些Joinpoint进行拦截的定义。真正增强的方法
Advice(通知/增强):所谓通知是指拦截到 Joinpoint 之后所要做的事情就是通知。
通知的类型:前置通知,正常返回通知,最终通知,环绕通知
Introduction(引介,了解):引介是一种特殊的通知在不修改类代码的前提下,Introduction 可以在运行期为类动态地添加一些方法或Field.
Target(目标对象):代理的目标对象
Proxy(代理):一个类被AOP织入增强后,就产生一个结果代理类
Weaving(织入):是指把增强应用到目标对象来创建新的对香港的过程。spring采用动态代理织入,而AspectJ采用编译期织入和类装载期植入
Aspect(切面):是切入点和通知(引介)的结合,描述了 增强具体应用的位置。
AOP的作用及优势
作用:在程序运行期间,在不修改源代码的情况下对方法进行功能增强。体现了java语言的动态性(反射)
优势:减轻重复代码,提高开发效率,并且便于维护
Spring 实现转账案例
实现所需:
一个account表用于存储用户的信息和基本金额:
建立maven的项目模块文件:
注意:其中这里的工具类ConnectionUtil、TransactionUtil是后面做测试用的可以不用建立
entity:Accoun.java
package com.etime.entity;import java.io.Serializable;public class Account implements Serializable {private Integer id;private String name;private double money;public Account() {}public Account(Integer id, String name, double money) {this.id = id;this.name = name;this.money = money;}public Integer getId() {return id;}public void setId(Integer id) {this.id = id;}public String getName() {return name;}public void setName(String name) {this.name = name;}public double getMoney() {return money;}public void setMoney(double money) {this.money = money;}@Overridepublic String toString() {return "Account{" +"id=" + id +", name='" + name + '\'' +", money=" + money +'}';}
}
dao:AccountDao.java
package com.etime.dao;import com.etime.entity.Account;import java.sql.SQLException;public interface AccountDao {Account getByName(String name);int updateAccount(Account account);
}
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><build><plugins><plugin><groupId>org.apache.maven.plugins</groupId><artifactId>maven-compiler-plugin</artifactId><configuration><source>7</source><target>7</target></configuration></plugin></plugins></build><groupId>com.etime</groupId><artifactId>day05</artifactId><version>1.0-SNAPSHOT</version><properties><spring.version>5.2.5.RELEASE</spring.version></properties><dependencies><!--导入spring的context坐标--><dependency><groupId>org.springframework</groupId><artifactId>spring-context</artifactId><version>${spring.version}</version></dependency><!--导入Jdbc模块依赖--><dependency><groupId>org.springframework</groupId><artifactId>spring-jdbc</artifactId><version>${spring.version}</version></dependency><!-- DBUtils --><!-- <dependency>--><!-- <groupId>commons-dbutils</groupId>--><!-- <artifactId>commons-dbutils</artifactId>--><!-- <version>1.6</version>--><!-- </dependency>--><!-- 数据库相关 --><!-- <dependency>--><!-- <groupId>mysql</groupId>--><!-- <artifactId>mysql-connector-java</artifactId>--><!-- <version>5.1.6</version>--><!-- </dependency>--><dependency><groupId>mysql</groupId><artifactId>mysql-connector-java</artifactId><version>8.0.11</version></dependency><!--c3p0--><dependency><groupId>com.mchange</groupId><artifactId>c3p0</artifactId><version>0.9.5</version></dependency><dependency><groupId>junit</groupId><artifactId>junit</artifactId><version>4.12</version><scope>test</scope></dependency><!-- 添加测试依赖--><dependency><groupId>org.springframework</groupId><artifactId>spring-test</artifactId><version>${spring.version}</version></dependency><!-- dbutils依赖的添加--><dependency><groupId>commons-dbutils</groupId><artifactId>commons-dbutils</artifactId><version>1.7</version></dependency><!-- 添加aop配置依赖--><dependency><groupId>org.aspectj</groupId><artifactId>aspectjweaver</artifactId><version>1.8.7</version></dependency></dependencies>
</project>
SpringConfig.java
package com.etime.util;import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.EnableAspectJAutoProxy;
import org.springframework.context.annotation.Import;//指定当前类的配置类相当于application.xml
@Configuration
@Import(DataSourceConfig.class)//导入连接数据
@ComponentScan("com.etime")//扫描文件
//@EnableAspectJAutoProxy//开启spring对注解AOP的支持
public class SpringConfig {
}
jdbc.properties
jdbc.driver=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/db_school?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=UTC
jdbc.username=root
jdbc.password=h123456
DataSourceConfig.java
package com.etime.util;import com.mchange.v2.c3p0.ComboPooledDataSource;
import org.apache.commons.dbutils.QueryRunner;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.PropertySource;
import org.springframework.jdbc.core.JdbcTemplate;import javax.sql.DataSource;
import java.beans.PropertyVetoException;
import java.sql.Connection;
import java.sql.SQLException;@PropertySource("classpath:jdbc.properties")
public class DataSourceConfig {@Value("${jdbc.driver}")private String driverClass;@Value("${jdbc.url}")private String jdbcUrl;@Value("${jdbc.username}")private String user;@Value("${jdbc.password}")private String password;public DataSource getDataSource(){ComboPooledDataSource ds= new ComboPooledDataSource();try {ds.setDriverClass(driverClass);ds.setJdbcUrl(jdbcUrl);ds.setUser(user);ds.setPassword(password);} catch (PropertyVetoException e) {e.printStackTrace();}return ds;}@Bean(name="jdbcTemplate")public JdbcTemplate getJdbcTemplate(){return new JdbcTemplate(getDataSource());}// @Bean(name ="connection")
// public Connection getConnection() throws SQLException {
// return getDataSource().getConnection();
// }
//
// @Bean(name = "queryRunner")
// public QueryRunner getQueryRunner(){
// return new QueryRunner();
// }
}
dao.impl:AccountDaoImpl.java
package com.etime.dao.impl;import com.etime.dao.AccountDao;
import com.etime.entity.Account;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanHandler;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.BeanPropertyRowMapper;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;import java.sql.Connection;
import java.sql.SQLException;@Repository("ad")
public class AccountDaoImpl implements AccountDao {@Autowiredprivate JdbcTemplate jdbcTemplate;
// @Autowired
// private Connection connection;
//
// @Autowired
// private QueryRunner queryRunner;public Account getByName(String name){Account account=null;String sql="select * from account where name=?";account=jdbcTemplate.queryForObject(sql,new BeanPropertyRowMapper<>(Account.class),name);return account;}public int updateAccount(Account account){int rows=0;String sql="update account set money=? where id=?";rows=jdbcTemplate.update(sql,account.getMoney(),account.getId());return rows;}
}
service:AccountService.java
package com.etime.service;import java.sql.SQLException;public interface AccountService {void transferAccount(String name1,String name2,double money);
}
service:AccountServiceImpl.java
package com.etime.service.impl;import com.etime.dao.AccountDao;
import com.etime.entity.Account;
import com.etime.service.AccountService;
import com.etime.util.TransactionUtil;
import org.apache.commons.dbutils.QueryRunner;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;import java.sql.SQLException;@Service("as")
public class AccountServiceImpl implements AccountService {@Autowiredprivate AccountDao accountDao;// @Autowired
// private TransactionUtil transactionUtil;//这里不能将此处的异常try,catch.spring只能捕捉throws的异常@Overridepublic void transferAccount(String name1, String name2, double money){
// try {//开启事务//transactionUtil.startTransaction();//收到的钱Account accountOne=accountDao.getByName(name1);accountOne.setMoney(accountOne.getMoney()+money);accountDao.updateAccount(accountOne);//钱转出Account accountTwo=accountDao.getByName(name2);accountTwo.setMoney(accountTwo.getMoney()-money);accountDao.updateAccount(accountTwo);//以上数据没有数据操作错误,就提交//transactionUtil.commitTransaction();
// }catch (SQLException e){
// //如果数据有误,进行数据回滚
// //transactionUtil.rollBackTransaction();
// e.printStackTrace();
// }finally {
// //如果服务结束,事务关闭(不管是否服务成功都进行最后的事务关闭)
// //transactionUtil.closeTransaction();
// }}
}
测试转账业务
正常的数据测试
AccountDemo.java
package com.etime.demo;import com.etime.service.AccountService;
import com.etime.util.SpringConfig;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;import java.sql.SQLException;@RunWith(SpringJUnit4ClassRunner.class)
//注意文件的配置,莫要写错
@ContextConfiguration(classes = {SpringConfig.class})
//@ContextConfiguration(locations = {"classpath:application.xml"})
public class AccountDemo {@Autowiredprivate ApplicationContext context;@Testpublic void t01(){AccountService accountService=(AccountService)context.getBean("as");accountService.transferAccount("hh","hu",30000);}
}
运行结果:
没有事务异常的情况下正常运行:
数据异常会有事务异常需要处理,并且数据前后需要一致性:
如图的转账明显不合理,本来没有金额,还把金额加上
处理事务问题
在DataSourceConfig中添加QueryRunner对象的初始化以及Connection对象的初始化
DataSourceConfig.java
package com.etime.util;import com.mchange.v2.c3p0.ComboPooledDataSource;
import org.apache.commons.dbutils.QueryRunner;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.PropertySource;
import org.springframework.jdbc.core.JdbcTemplate;import javax.sql.DataSource;
import java.beans.PropertyVetoException;
import java.sql.Connection;
import java.sql.SQLException;@PropertySource("classpath:jdbc.properties")
public class DataSourceConfig {@Value("${jdbc.driver}")private String driverClass;@Value("${jdbc.url}")private String jdbcUrl;@Value("${jdbc.username}")private String user;@Value("${jdbc.password}")private String password;public DataSource getDataSource(){ComboPooledDataSource ds= new ComboPooledDataSource();try {ds.setDriverClass(driverClass);ds.setJdbcUrl(jdbcUrl);ds.setUser(user);ds.setPassword(password);} catch (PropertyVetoException e) {e.printStackTrace();}return ds;}// @Bean(name="jdbcTemplate")
// public JdbcTemplate getJdbcTemplate(){
// return new JdbcTemplate(getDataSource());
// }@Bean(name ="connection")public Connection getConnection() throws SQLException {return getDataSource().getConnection();}@Bean(name = "queryRunner")public QueryRunner getQueryRunner(){return new QueryRunner();}
}
编写事务管理工具
TransactionUtil是管理事务的工具类,主要定义了和事务相关的方法,事务开启,事务提交,事务回滚等。该工具目前需要手动创建和管理,使用Spring进行事务管理后,该工具类由Spring提供,不需要手动编写和管理。所以该工具类重在理解,为Spring进行事务管理做好铺垫
TransactionUtil.java
package com.etime.util;import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.*;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;import java.sql.Connection;
import java.sql.SQLException;@Component("transactionUtil")
//声明为切面
@Aspect
public class TransactionUtil {@Autowiredprivate Connection connection;
//
// @Pointcut("execution(public void com.etime.service.impl.AccountServiceImpl.transferAccount(..))")
// private void point(){}
//
// //把当前方法看成是前置通知
// //@Before("execution(public void com.etime.service.impl.AccountServiceImpl.transferAccount(..))")
@Before("point()")public void startTransaction(){try {System.out.println("开启事务");connection.setAutoCommit(false);} catch (SQLException e) {e.printStackTrace();}}
//
// //把当前方法看作是后置通知
// //@AfterReturning("execution(public void com.etime.service.impl.AccountServiceImpl.transferAccount(..))")
// //@AfterReturning("point()")public void commitTransaction(){try {System.out.println("事务提交");connection.commit();} catch (SQLException e) {e.printStackTrace();}}
//
// //把当前方法看作是异常通知
// //@AfterThrowing("execution(public void com.etime.service.impl.AccountServiceImpl.transferAccount(..))")
// //@AfterThrowing("point()")public void rollBackTransaction(){try {System.out.println("事务回滚");connection.rollback();} catch (SQLException e) {e.printStackTrace();}}
//
// //把当前的方法看作是运行结束通知的方法
// //@After("execution(public void com.etime.service.impl.AccountServiceImpl.transferAccount(..))")
// //@After("point()")public void closeTransaction(){try {System.out.println("释放资源");connection.close();} catch (SQLException e) {e.printStackTrace();}}
//
// //环绕通知方法
// //把当前方法看成是环绕通知
// //@Around("execution(public void com.etime.service.impl.AccountServiceImpl.transferAccount(..))")
// @Around("point()")
// public Object transactionAround(ProceedingJoinPoint pjp){
// Object result = null;
// try {
// //获取调用切入点方法时传入的参数
// Object[] args = pjp.getArgs();
// //启动事务
// startTransaction();
// //运行切入点方法
// result = pjp.proceed(args);
// commitTransaction();
// } catch (Throwable e) {
// //运行有误需要回滚
// rollBackTransaction();
// e.printStackTrace();
// }finally {
// //运行结束释放资源
// closeTransaction();
// }
// return result;
// }
}
dao:AccountDao.java
package com.etime.dao;import com.etime.entity.Account;import java.sql.SQLException;public interface AccountDao {Account getByName(String name) throws SQLException;int updateAccount(Account account) throws SQLException;
}
dao:AccountDaoImpl.java
package com.etime.dao.impl;import com.etime.dao.AccountDao;
import com.etime.entity.Account;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanHandler;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.BeanPropertyRowMapper;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;import java.sql.Connection;
import java.sql.SQLException;@Repository("ad")
public class AccountDaoImpl implements AccountDao {
// @Autowired
// private JdbcTemplate jdbcTemplate;@Autowiredprivate Connection connection;@Autowiredprivate QueryRunner queryRunner;public Account getByName(String name) throws SQLException {Account account=null;String sql="select * from account where name=?";account=queryRunner.query(connection,sql,new BeanHandler<>(Account.class),name);return account;}public int updateAccount(Account account) throws SQLException {int rows=0;String sql="update account set money=? where id=?";rows=queryRunner.update(sql,account.getMoney(),account.getId());return rows;}
}
service:AccountService.java
package com.etime.service;import java.sql.SQLException;public interface AccountService {void transferAccount(String name1,String name2,double money);
}
service:AccountServiceImpl.java
package com.etime.service.impl;import com.etime.dao.AccountDao;
import com.etime.entity.Account;
import com.etime.service.AccountService;
import com.etime.util.TransactionUtil;
import org.apache.commons.dbutils.QueryRunner;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;import java.sql.SQLException;@Service("as")
public class AccountServiceImpl implements AccountService {@Autowiredprivate AccountDao accountDao;@Autowiredprivate TransactionUtil transactionUtil;//这里不能将此处的异常try,catch.spring只能捕捉throws的异常@Overridepublic void transferAccount(String name1, String name2, double money){try {//开启事务transactionUtil.startTransaction();//收到的钱Account accountOne=accountDao.getByName(name1);accountOne.setMoney(accountOne.getMoney()+money);accountDao.updateAccount(accountOne);//钱转出Account accountTwo=accountDao.getByName(name2);accountTwo.setMoney(accountTwo.getMoney()-money);accountDao.updateAccount(accountTwo);//以上数据没有数据操作错误,就提交transactionUtil.commitTransaction();}catch (SQLException e){//如果数据有误,进行数据回滚transactionUtil.rollBackTransaction();e.printStackTrace();}finally {
// //如果服务结束,事务关闭(不管是否服务成功都进行最后的事务关闭)transactionUtil.closeTransaction();}}
}
测试:
AccountDemo.java
package com.etime.demo;import com.etime.service.AccountService;
import com.etime.util.SpringConfig;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;import java.sql.SQLException;@RunWith(SpringJUnit4ClassRunner.class)
//注意文件的配置,莫要写错
@ContextConfiguration(classes = {SpringConfig.class})
//@ContextConfiguration(locations = {"classpath:application.xml"})
public class AccountDemo {@Autowiredprivate ApplicationContext context;@Testpublic void t01(){AccountService accountService=(AccountService)context.getBean("as");accountService.transferAccount("hh","hu",30000);}
}
运行结果:
处理了事务,如果遇到抛出问题,数据库进行操作的不满足加连之前的操作全部回滚,这样才满足数据操作前后一致性。
查看全文
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如若转载,请注明出处:http://www.dgrt.cn/a/2272970.html
如若内容造成侵权/违法违规/事实不符,请联系一条长河网进行投诉反馈,一经查实,立即删除!
相关文章:
SpringAOP入门基础银行转账实例————事务处理
SpringAOP入门基础银行转账实例————事务处理
AOP为Aspect Oriented Programming 的缩写,意思为面向切面编程,是通过编译方式和运行期动态代理实现程序功能的统一维护的一种技术。
AOP编程思想
AOP面向切面是一种编程思想,是oop的延……
Leetcode203.removeElements 移除链表元素
目录
前言
一、原题说明与链接
二、思路 判断当前链表的节点值是否等于val,如果不等于,拿下来尾插,循环判断,直到原链表为空
2.1调试创建一个单链表
2.2移除元素
总结 前言
本文记录了这道题目的题解方法与思路及调试情况&……
Latex {multicols}{2}环境下插入单栏/双栏表格
背景
期刊模板中未给出表格插入,因此需要单双栏插入方式汇总。相比于图片和公式,此部分细节或者试错次数更多。 已经可以轻易地搜到许多教程,熟悉基本语法之后,若普通的功能满足不了需求,则这里可能提供相应的解决方式……
05 | HTTP调用:你考虑到超时、重试、并发了吗?
HTTP调用:你考虑到超时、重试、并发了吗?
进行 HTTP 调用本质上是通过 HTTP 协议进行一次网络请求,需要考虑以下三点
框架设置的默认超时是否合理;考虑到网络的不稳定,超时后的请求重试是一个不错的选择,……
java模拟长连接发送
1、客户端
package com.msb.netty.testPageLongKeepAlive;import java.io.IOException;
import java.io.InputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.net.Socket;
import java.net.UnknownHostException;
import java.ut……
【ChatGPT】Prompt Engineering入门
Prompt Engineering入门一、什么是 Prompt Engineering?二、我们还需要学习 PE 吗?三、Prompt基础原则一、什么是 Prompt Engineering? 简单的理解它是给 AI 模型的指令。它可以是一个问题、一段文字描述,甚至可以是带有一堆参数的……
【Python学习笔记】1. Python数据容器
1. Python数据容器1.1 列表(list)1.1.0 相关函数与方法总览1.1.1 列表的定义与下标访问1.1.2 列表的查找、修改、插入、追加1.1.3 列表的删除、弹出、清空1.1.4 列表元素数量的统计1.1.5 列表的遍历(while与for)1.2 元组ÿ……
【算法训练 (day2)】积木画(dp问题)
一.问题
题目描述
小明最近迷上了积木画,有这么两种类型的积木,分别为 I 型(大小为 2 个单位面积)和 L 型(大小为 3 个单位面积): 同时,小明有一块面积大小为 2 N 的画布&#……
每日一问—03如何利用华为云和Frp进行内网穿透
1、部署华为云安全组 2、登陆服务器,部署服务器端
(1)frp开源地址:Releases fatedier/frp (github.com)
(2)下载解压
wget https://github.com/fatedier/frp/releases/download/v0.43.0/frp_0.43.0_linux_amd64.tar.gztar -z……
景点VR全景虚拟体验系统定制
为深度挖掘行业特色,利用5G、VR,AI,AR等数字化技术,为行业领域量身打造数字化解决方案已成趋势 VR内容定制可包括: VR旅游、VR展馆、VR教育、VR汽车、VR电商、VR地产等等。我们是国内较早从事沉浸式VR内容开发的企业,在……
quick-cocos2dx lua语言讲解 (动作,定时器,触摸事件,工程的类的讲解)
–MainScene部分
— display.newScene 创建一个场景 — 在quick里面我们的控件、精灵 一般是加载到场景上的 local MainScene class("MainScene", function() return display.newScene("MainScene") end) function MainScene:ctor() –创……
使用quick-cocos2dx-lua 实现的小游戏(包含碰撞检测,触屏发子弹)
–主界面local MainScene class("MainScene", function()return display.newScene("MainScene")end)ON true;function MainScene:ctor()local bg cc.Sprite:create("main_background.png");bg:setScale(2);bg:setPosition(display.cx,display……
cocos2d-js 中scrollview详解
/****
开头的一些废话:
1、多思考,善于思考
2、懂得变通
3、多多查询API首先复制一段 API中的源码:(UIScrollView.js)这段代码可以看出 scrollview
中的容器是一个node,并且他的位置是:代码最后……
cocos2d-js中的回调函数中世界坐标系和节点坐标系的相互转换
世界坐标系和节点坐标系都是OPENGL 坐标系 1、世界坐标系原点就是屏幕的左下角; 2、节点坐标系的原点就是一个节点的左下角; 3、两个坐标系可以通过已经写好的cocosAPI进行想换转换; 4、所有的节点需要转为一个节点上或者是统一的世界坐标系……
通过JavaScript实现漂浮
<html>
<head><meta http-equiv"Content-Type" content"text/html"; charset"gb2312" /><title>漂浮广告</title><style type"text/css">div{position:absolute;}</style>
</head>
&……
序列动画和图片内存问题
一、帧动画问题 /*** 帧动画总结:* 1、如果精灵进行新建时,加载了纹理,那么setRestoreOriginalFrame可以设置为false或者true* 2、如果精灵新建时,没有加载纹理的话,那么setRestoreOriginalFrame需要设置为false&#……
冒泡排序的两种写法
//第一种写法: #include <iostream> using namespace std; void bubbleSort(int arr[], int n) { for (int i 0; i < n-1; i) { int temp 0; for (int j i1; j <n; j) { if (arr[i] ……
c++中this详解
http://blog.csdn.net/ugg/article/details/606396…
编程是可以从事一生的职业吗
暂且不论这个话题,留待以后再添加见解。
目前而言,编程符合自己的性格和兴趣,且可以解决自我的生活,一举两得,总算是做了自己喜欢的事情。2016-7-24
在此立文以自勉!
2016-8-30: 1、编程就是一个不断和自我的惰性做……
STL中常用容器详解
常用的容器
一、顺序容器 1、vector 向量 :随机访问(按照下标)任何一个元素,在尾部增删元素,相当于是一个动态的数组。 vector容器,在头部增加、删除元素,其时间消耗和元素数目成正比ÿ……
编程日记2023/4/16 15:01:19